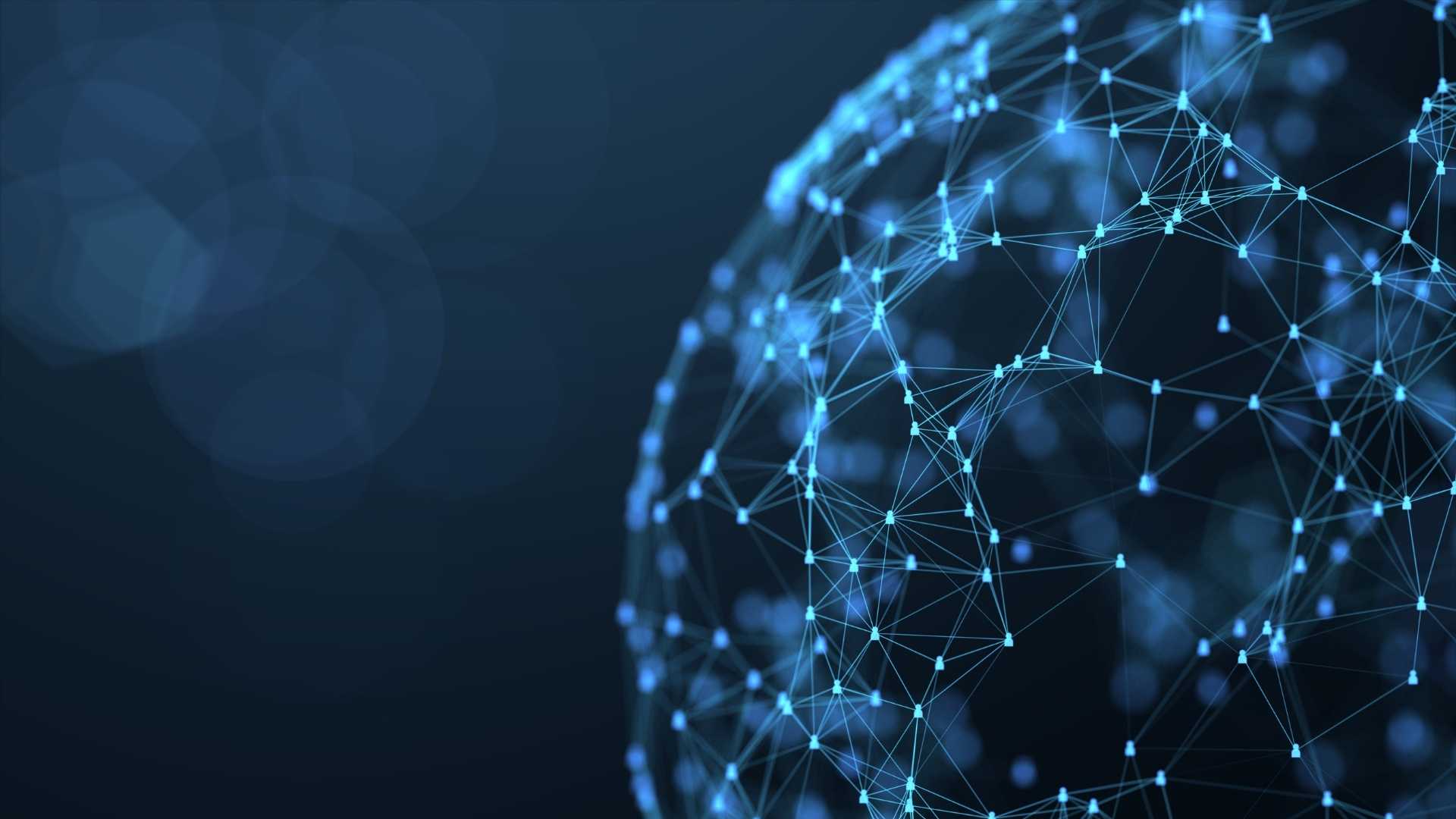
Toolbox: DataFrame points to GeoSeries
Tutorial presents how to transform points as floats written in DataFrame
into GeoSeries
object.
Updates
- 2021-06-28: GeoPandas method
geopandas.points_from_xy
included in the tutorial due to the nice response from GeoPandas developers on Twitter (here).
Requirements
pandas
to readcsv
files with float coordinates,geopandas
to createGeoSeries
.
import geopandas as gpd import pandas as pd
Proof of Concept
GeoPandas allows us to transform any list of points into Point() with geopandas.points_from_xy()
method:
# Transformation of floats to the Point lat = 51.5074 lon = 0.1278 point = gpd.points_from_xy([lat], [lon])
Function
# Transform sample def lat_lon_to_point(dataframe, lon_col='longitude', lat_col='latitude', crs='WGS84'): """Function transform longitude and latitude coordinates into GeoSeries. INPUT: :param dataframe: DataFrame to be transformed, :param lon_col: (str) longitude column name, default is 'longitude', :param lat_col: (str) latitude column name, default is 'latitude', :param crs: (str) crs of the output geoseries. OUTPUT: :return: (GeoPandas GeoSeries object) """ geometry = gpd.points_from_xy(dataframe[lon_col], dataframe[lat_col]) geoseries = gpd.GeoSeries(geometry) geoseries.name = 'geometry' geoseries.crs = crs return geoseries
The important thing is that longitude is x
coordinate and latitude is y
coordinate. We must set coordinate reference system (CRS) to create valid GeoSeries
. In most cases CRS of point measurements is WGS84 popularized by the Global Positioning Systems.
You may now work with your GeoSeries
and use spatial methods and attributes of this object!
Requirements – old algorithm
pandas
to readcsv
files with float coordinates,geopandas
to createGeoSeries
,shapely
to transform floats intoPoint
.
import geopandas as gpd import pandas as pd from shapely.geometry import Point
Proof of Concept – old algorithm
Two floats may be easily transformed into Point
with shapely
. List or tuple of two floats is passed into the Point
class constructor:
# Transformation of floats to the Point lat = 51.5074 lon = 0.1278 sample_coordinate = [lon, lat] # x, y point = Point(sample_coordinate)
Function – old algorithm
The hardest part of transformation is to transform DataFrame
float columns at once. We use for it .apply()
method and lambda
function.
# Transform float columns into geoseries def lat_lon_to_point(dataframe, lon_col='longitude', lat_col='latitude', crs='WGS84'): """Function transform longitude and latitude coordinates into GeoSeries. INPUT: :param dataframe: DataFrame to be transformed, :param lon_col: (str) longitude column name, default is 'longitude', :param lat_col: (str) latitude column name, default is 'latitude', :param crs: (str) crs of the output geoseries. OUTPUT: :return: (GeoPandas GeoSeries object) """ geometry = dataframe.apply(lambda x: Point([x[lon_col], x[lat_col]]), axis=1) geoseries = gpd.GeoSeries(geometry) geoseries.name = 'geometry' geoseries.crs = crs return geoseries